Wix Blocks Tutorial: Creating a Countdown Widget
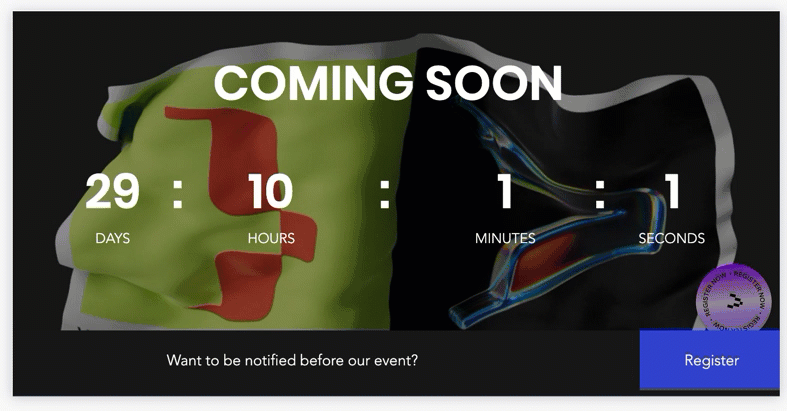
What you get in the template
- A countdown widget. You need to complete its design and code its functions and properties.
- A registration widget which you need to complete and add to your countdown widget.
- Some public utilities that you can use to implement your widget's functionality.
- Some backend code that you can use to implement the functions of your email notification.
Step 1 | Complete the Design of Your Widget
- Open the template.
- Click Countdown under Widgets and Design.
- Click Add Elements
in the top bar.
- Select Text. Drag and drop a 48px Title box into your widget.
- Click Edit Text and change the text to SS.
- Change the text color to white and center the text using the Inspector
.
- Select the text box and move it into the grid square above the SECONDS label using
.
- Resize it using
.
Step 2 | Add and Define Your Widget's API Properties
- endDate: Defines the end date when the countdown reaches zero. Using this property, site creators can change the end date so that the widget is customized for their site.
- emailId: Determines the email message that will be sent to users when they sign up for a notification.
Give your widget elements an ID
- Click on the text box.
- Give the text box an ID in the Properties & Events
panel. In this example the ID is
secondsTxt
. Note that the other elements have already been give IDs.
Define the "endDate" and "emailId" properties
- Click Add New Property in the API
panel.
- Enter the property name,
endDate
. - Select the property type, in this case, Date and Time (the date is displayed in US format mm/dd/yyyy).
- Select a default value (the site creator will be able to change this when they install your widget in the editors).
- Hover over Properties and click the
icon that appears.
- Create another property for the triggered email and call it
emailId
. This is a Text type property with no default value.
Step 3 | Add Code to Make Your Widget Work

Import your utilities
- Click on the Public & Backend
menu.
- Select dateUtils.js under Public. This opens the file in the code panel.
Change the code to consider your new function
Create a function, updateTime()
, so that your countdown widget will update the count. Your new function should look like this:
1$widget.onPropsChanged(function () {
2});
3 function updateTime() {
4 const {days, hours, minutes, seconds} = getRemainingTime (new Date($widget.props.endDate));
5 $w('#daysTxt').text = days.toString();
6 $w('#hoursTxt').text = hours.toString();
7 $w('#minutesTxt').text = minutes.toString();
8 $w('#secondsTxt').text = seconds.toString();
9 }
You also need to set an interval for how often the widget updates the remaining time.
To do this, add the following code in your onReady()
function. This updates the widget every second.
1$w.onReady(function () {
2 updateTime();
3 if (wixWindow.viewMode !== "Editor") {
4 setInterval(updateTime, 1000);
5 }
6});
Preview your widget and test its API properties
- Click Preview. Your widget should countdown every second to the end date.
- Click Test API Properties. A panel appears with the default value you set earlier.
- Change the date to check if the widget responds and counts down to the new date.
Step 4 | Create and Code a Custom Panel
Create a custom panel
- Click on the Panels tab in the top bar.
- Click Create Panel.
- Name your panel. In this example, "My settings".
- Click Create Panel.
- Click + Add Element.
- Select Text Input. This will be for the end date.
- Click Properties & Events
. Give your text input an ID -
endDateInput
. - Select the text element and click Settings.
- Add the name "End Date" in the Field Title field.
- Delete the text in the Default Text field.
- Set the placeholder text to "End Date".
- Add a section divider.
- Click Settings. Add a title in the Section Title field. In this example, "Triggered email".
- Add another Text Input. Give your text input an ID -
emailIdInput
. - Select the text element and click Settings.
- Add the name "Email ID" in the Field Title field.
- Delete the text in the Default Text field.
- Set the placeholder text to "Email ID".
Add code to your custom panel
To enable panel elements to interact with your widget and perform actions in the editor, you can use the Velo wix-widget
module in your code.
To use the Widget API, import wixWidget
from the wix-widget
module.
Insert the following code before your onReady()
function.
1import wixWidget from 'wix-widget';
You want your widget to update when the values change so you need to register an onChange
event. You also need to add async
in your onReady ()
function as you will be using some asynchronous functions.
Your onReady()
function should look like this:
1$w.onReady(async function () {
2 const { endDate, emailId } = await wixWidget.getProps();
3 $w('#endDateInput').value = endDate;
4 $w('#emailIdInput').value = emailId;
5
6 $w('#endDateInput').onChange(e => {
7 wixWidget.setProps({ endDate: e.target.value });
8 });
9
10 $w('#emailIdInput').onChange(e => {
11 wixWidget.setProps({ emailId: e.target.value });
12 });
13});

Step 5 | Configure Your Widget
Add your custom panel to your widget's floating action bar
- Click Configuration in the top bar.
- Select your countdown widget. A floating action bar appears.
- Click Settings.
- Click
Action Button Settings. The Main Action Settings panel appears.
- Select My settings from the dropdown list to select your custom panel.
Step 6 | Add a Second (Inner) Widget and Implement the Registration Logic
Add your registration widget to the countdown widget
- Working in the Design tab, select your countdown widget.
- Click More Options
and select Add Widget.
- Select Registration. Your inner widget appears in the middle of your countdown widget.
- Drag and stretch your innner widget to fit the lower section of your countdown widget.
- Change its ID to
registration
in Properties & Events.

Implement the registration logic in the main widget's code
You'll implement the code for user registeratiion in the main (countdown) widget, using the pre-installed utility, contactUtils.js
. It uses the wix-crm
API to connect your contact to your collection.
This code directs the information to your collection. It uses the utility you will use later when you create a collection. You can see getSubscriptionsCollectionName()
in the code.
- Create a new async function.
- Call it
onSubmit
. It uses the create contact utility.
Your code should look like this:
1 async function onSubmit({ email }) {
2 const contact = await createContact(email);
3 wixData.insert(getSubscriptionsCollectionName(), {
4 endDate: new Date($widget.props.endDate),
5 emailId: $widget.props.emailId,
6 contactId: contact.contactId
7 });
8 }
9
Register for the onSubmit event
In your onReady()
, after your updateTime()
function, add the following code:
1$w('#registration').onSubmit(onSubmit);
Step 7 | Build Your App and Give it a Namespace
Your first build
- Click Build.
- Enter a namespace for your app and click Next.
- Select Major Version and click Build.
- You get a message that Version 1.0 is built. Click Got it to continue working on your app.
Step 8 | Add a Collection to Your Widget
The template has a pre-installed utility called getSubscriptionsCollectionName()
, in collectionUtils.js under Public & Backend. It constructs the full name of the collection so that you don't have to add your full app namespace every time you refer to it in the code.
Configure your collection utility
- Click collectionUtils.js under Public & Backend.
- Add your app's namespace.
1
2const NAMESPACE = '@mywixaccount/my-app-namespace';
3
4export function getSubscriptionsCollectionName() {
5 return `${NAMESPACE}/subscriptions`;
6}
Create your collection
- Click Databases
- Click + Create collection
- Give your collection a meaningful name. In this example, Subscriptions.
- Click Create.
Add fields to your collection
- Click
to add a field to your collection.
- Select Date from the Field Type drop down menu.
- Enter endDate in the Field Name field. This will be the last date up until which people can register.
- Click Save.
- Now add a Text type field and call it emailId for the triggered email.
- Add another Text type field and call it contactId. This will store the contact details of registered users.
- Now create a Boolean type field and call it notified. This ensures that subscribers will be notified only once.
Set permissions for your collection
- Click More Options
for your collection from the Databases menu.
- Select Permissions & Privacy.
- Select the dropdown menu Can add content.
- Select Anyone.
- Click Save.
Step 9 | Install Your App on a Site in the Wix Editor
- After building your app, open your website.
- Click Add apps
.
- Click Custom Apps. A list of all your apps appears.
- Select your app from the Available apps list.
- Click Install App.
- Click Add Elements
.
- Select My Widgets.
- Double click your widget to add it to your site.
Set an Email ID
- Open My Dashboard from the Site menu in the top bar.
- Go to Triggered Emails in Developer Tools.
- Click Get Started.
- Enter a subject.
- Design the email using the editor tools.
- Click Save & Publish.
- Add the sender details, the From name and the Reply-to email and click Save.
- Click Got it.
- Click Save & Publish again. You get a generated identification code that links to the email that you have designed so that it will be sent to anyone who registers.
- Enter this code in your custom panel in the Email ID field.
Use Backend Code in Wix Editor to Notify Subscribers
Invoke your notify function
- Click + New web module under Backend in Public & Backend in the Wix editor.
- Call it
backend.jsw
. - Import the backend function in your site's code section under backend.jsw.
Your code should look like this:
1import { notify } 'myWixId/my-application-name-backend';
Now create an export function invokeNotify
in backend.jsw in your site's code section.
Your code should look like this:
1export function invokeNotify() {
2 return notify ();
3}
- Click Add
in Backend
- Click
Add scheduled jobs. Add the following code under jobs.config in your site's code section. In this example the notify function is invoked at 10 minutes past the hour, every hour.
1{
2 "jobs": [{
3 "functionLocation": "/backend.jsw",
4 "functionName": "invokeNotify",
5 "description": "",
6 "executionConfig": {
7 "cronExpression": "10 * * * *"
8 }
9 }]
10}
Step 10 | Test Your App
- Publish your site.
- Register to receive an email notification.
- Go back to the editor and check your collection.
- You can see there is a new subscriber. When the notification email is sent you will also see a tick in the notified field.