Wix Blocks: Adding Code to Your Custom Panels
5 min read
In this article
- Add code to your custom panel
- Panel elements
- Velo modules for Blocks custom panels
- Add code that interacts with your widget
- Add code that interacts with your panel
- Example using code that impacts the panel
Wix Blocks is open to all Wix Studio users. To get access to Blocks, join Wix Studio.
Blocks enables you to create custom panels for your action bars, so that when a site creator clicks an action bar button (for example, the Settings button) - a custom panel opens, instead of the default panel.
Creating your panel has two main parts. First, design your panel by adding its elements and customizing them. Then add code to your panel, to determine how it interacts with the widget or to control the behavior of the panel itself.
API reference for panel elements
See all panel elements API reference so you can work with them in your code. Note that this temporary preview of the Velo API reference is only open to the early users of Blocks.
Add code to your custom panel
- Make sure that you are in the Panels tab.
- Make sure that your panel is selected in the Panels section on the left menu (or create a new panel, if you haven't created it yet).
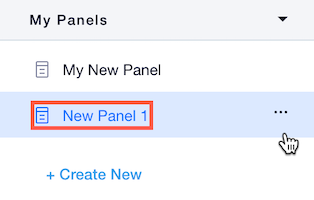
- Go to your panel's code section.
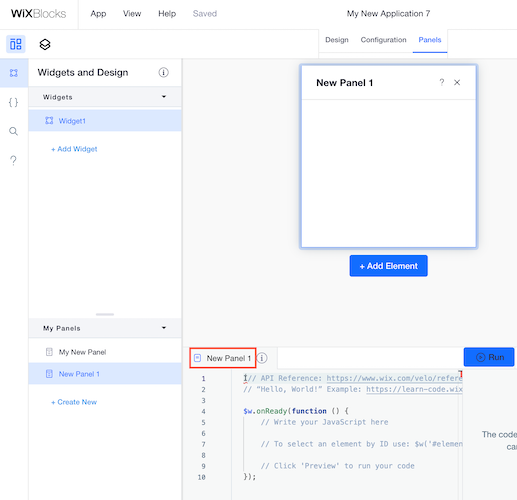
Panel elements
Custom panels are made of unique user interface (UI) elements, such as buttons, checkboxes, drop-down lists and more. See the list of elements and the Velo API reference for all panel elements, to work with them in your code. Note: this is a temporary view of API reference, only open for Blocks users. It takes about 20 seconds to lode.
Velo modules for Blocks custom panels
To enable panel elements to interact with your widget and perform actions in the editor, use one or more of the following modules in your panel code:
The wix-widget
module controls your widget's properties, design presets, and more.
1import wixWidget from 'wix-widget'
The wix-editor
module interacts with the Editor to remove or restore widget elements, open Dashboard panels, and more.
1import wixEditor from 'wix-editor'
Add code that interacts with your widget
Write whatever you want to happen in the onReady function of your panel's code. For example, in a shopping widget, you can turn a "special sale" property on and off, through a toggle element in the widget's props.
1import wixWidget from 'wix-widget';
2
3$w.onReady(async function () {
4 $w(“#panelToggleSwitch1”).onChange(async () => {
5 const props = await wixWidget.getProps();
6 if (props.saleIndicator === "Sale") {
7 await wixWidget.setProps({ saleIndicator: "No Sale" });
8 } else {
9 await wixWidget.setProps({ saleIndicator: "Sale" });
10 }
11 });
12});
Here is a way for showing and hiding an element through the panel code.
1import wixEditor from 'wix-editor';
2
3$w.onReady(async function () {
4 $w('#toggleBox').onClick(async (event: any) => {
5 if (event.target.value) {
6 await wixEditor.removeElement('#title');
7 } else {
8 await wixEditor.restoreElement('#title');
9 }
10 });
11});
Here is an example of changing the widget's design preset from the panel code. The panel has three buttons, for three different presets, and looks like this:
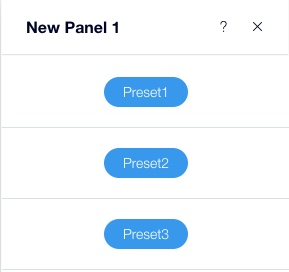
Here is the panel's code:
1import wixWidget from 'wix-widget'
2
3$w.onReady(async function () {
4 $w("#panelButton1").onClick(async () => {
5 await wixWidget.setDesignPreset("Preset1")
6 })
7
8 $w("#panelButton2").onClick(async () => {
9 await wixWidget.setDesignPreset("Preset2")
10 })
11
12 $w("#panelButton3").onClick(async () => {
13 await wixWidget.setDesignPreset("Preset3")
14 })
15
16 const currentDesignPreset = await wixWidget.getDesignPreset();
17 console.log('currentPreset', currentDesignPreset);
18
19})
Add code that interacts with an inner (nested) widget
This example shows how to set a "Sale" or "No Sale" tag in the widget props, but for an inner (nested) widget.
1$w.onReady(async function () {
2 const innerWidget = await wixWidget.getNestedWidget("#nestedWidget1");
3 $w(“#panelToggleSwitch1”).onChange(async () => {
4 const innerProps = await innerWidget.getProps();
5 if (innerProps.saleIndicator === "Sale") {
6 await innerWidget.setProps({ saleIndicator: "No Sale" });
7 } else {
8 await innerWidget.setProps({ saleIndicator: "Sale" });
9 }
10 });
11});
Add code that interacts with your panel
In some cases, you might want to manipulate what happens within the panel itself. For example, you might want some of the panel elements to be hidden if they are irrelevant (if an item is out of stock, for example, there are some choices that you don't need in the panel).
In the following example, a toggle determines whether a checkbox group is shown or collapsed.
1$w.onReady(async function () {
2 $w(“#wbuToggleSwitch1”).onChange(async () => {
3 if ($w(“#panelCheckboxGroup1”).collapsed) {
4 await $w(“#panelCheckboxGroup1").expand();
5 } else {
6 await $w(“#panelCheckboxGroup1”).collapse();
7 }
8 });
9})
Connecting your panel to a button
When you have added code to your panel you are ready to connect it to an action bar button. Learn more
Test your panel across editors
We recommend that you install your widget both on a Wix Editor and an Editor X site, to check how the panels work.
Example using code that impacts the panel
This panel for the Login Bar Settings button has a toggle, which determines whether or not to show a greeting before the user's name.
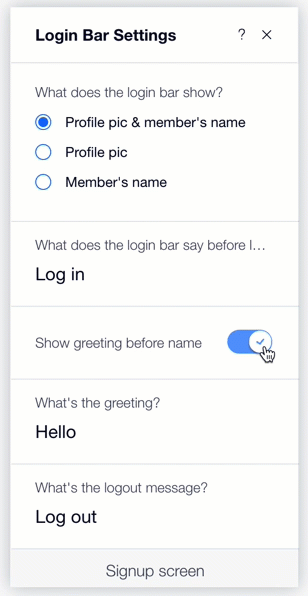
To make your toggle switch work:
- Select the toggle switch element.
- Change the ID to a meaningful name in Properties & Events
The toggle switch here is called
showGreetingSwitch
. - Change the ID of the text box element. Here it is called
greetingText
. - Add the following code to your panel's
onReady()
function:
1$w.onReady(function () {
2 $w('#showGreetingSwitch').onChange((event) => {
3 if (event.target.checked) {
4 $w('#greetingText').expand();
5 }
6 else {
7 $w('#greetingText').collapse();
8 }
9 })
10});