Wix Blocks: Widget API Functions
2 min read
Wix Blocks is currently open to a limited number of users.
Add a new function to enable site creators to perform actions related to the widgets on their websites. For example, a site creator who has a shopping widget would like to be able to get the address of a certain customer through the function getAddress(customerId) and set the address through the function setAddress(customerId).
To add a new public function to your widget:
1. Click the Widget API
icon.

2. Click Add New Public Function or hover over Functions and click the
icon.

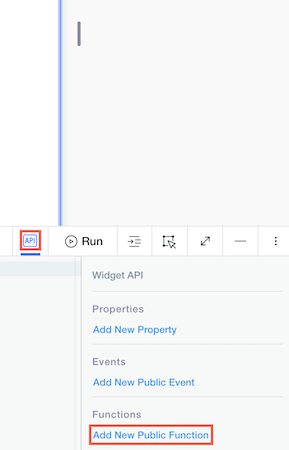
3. Add the code for your function in the code panel, where a new function "foo" appears.
4. Change the new default name to a meaningful name and edit the function.
1import {getJSON} from 'wix-fetch';
2
3/**
4*@function
5*@description Add item to cart
6*@param {string} productId - The Id of the product to add to the cart
7*@param {number} quantity - How many products to add
8*@returns {Promise<boolean>} True on success, false on error
9*/
10export async function addItemToCart(productId, quantity){
11 try {
12 const addToCartReponse = await getJSON('https://myCustomStoreService/cart', {
13 method: 'POST',
14 body: JSON.stringify({
15 productId,
16 quantity,
17 })
18 })
19
20 return addToCartReponse.success
21 } catch (e) {
22 return false
23 }
24}
Don't forget to document
When you write your function, make sure to replace the automatic jsdoc that appears above it with your own one. The user of the widget will see the jsdoc when using the function in a site.
Next Step: Using the Widget API on a Site
Want to learn more about Blocks?
Check out the Wix Blocks help articles
Did this help?
|