Tutorial: Creating a Package for API Calls
6 min
All Wix users can create Velo Packages. To create apps for Wix sites with the full version of Wix Blocks, join Wix Studio.
This tutorial walks you through the process of creating a code package that includes the frontend and backend Velo code for an API call. The code retrieves stock prices from https://iexcloud.io/. You could put all of the code in the frontend, but like many APIs, this one requires a key, and it's insecure to expose your key in frontend code.
To complete this tutorial, you'll need a key, which you can get when you register at https://iexcloud.io/cloud-login#/register.
This package provides:
- The frontend code that enables the user to input a stock symbol and receive the latest price, and a message as to whether the market is open or closed.
- The backend code that retrieves the key from the Velo Secrets Manager and assembles the URL for the API request, including the stock symbol and the key.
This tutorial also describes how to use this package on a Wix site.
Step 1 | Open Wix Blocks for Package Creation
- Turn on Dev Mode in any Editor or Editor X site.
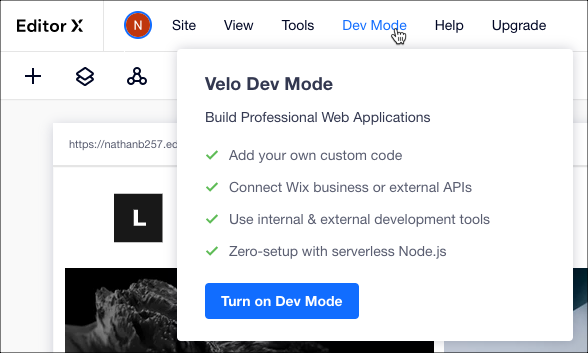
- Open Code Files from the left panel.
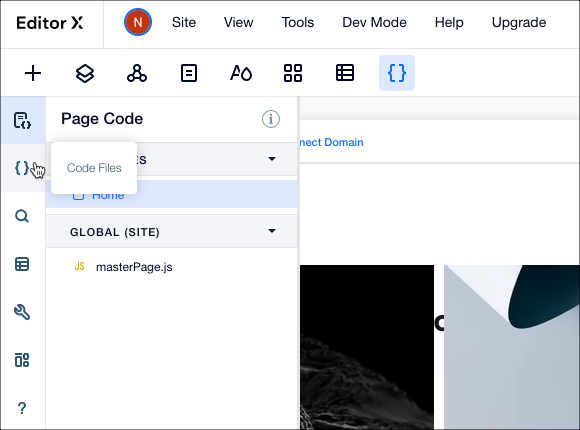
- Click the More Actions icon in the Package section and click Create a Package.
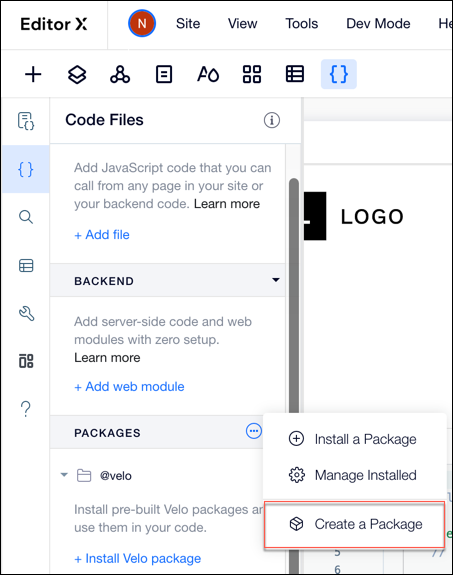
- Click Get Started on the Create Your Own Code Package panel. This opens Wix Blocks in a new browser window.
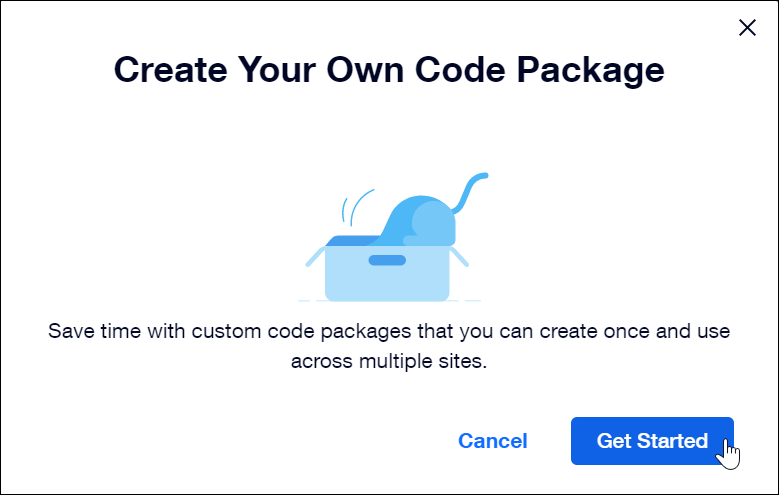
Step 2 | Add Code Files to Your Package
- Add a public code file for the frontend code in Wix Blocks.
- Select Code Files.
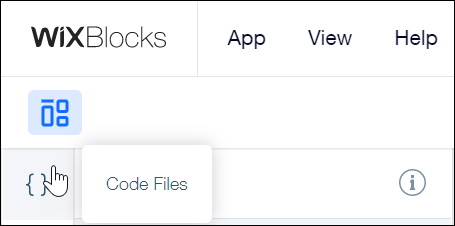
b. Click the
icon and select New JS File in the PUBLIC section. c. Name the file stockInfo.js.
d. Paste this code in the code file panel that opens. The code calls the backend function that retrieves the stock information, then displays the results on the web page.
1import { getStockData } from 'backend/apiCall.jsw';
2
3export async function getStock()
4{
5 const quote = await getStockData()
6 $w('#latestPrice').text = quote.latestPrice.toString()
7 if (quote.isUSMarketOpen === "true") {
8 console.log("Market is open, price is :",quote.latestPrice)
9 } else {
10 console.log("Market is closed, price is :",quote.latestPrice)
11 }
12}
2. Add a config.js file to store a default stock symbol for your site. If the user provides spaces instead of a stock symbol, the quote for the default symbol is displayed. Because the config.js file is editable on your website, you can change the default symbol on each site.
a. Select the config.json file under BACKEND > CONFIGURATION
b. Copy this code into the file, using any stock symbol you choose. The stock symbol is editable for each site.
1{
2 "defaultSymbol": "wix"
3}
3. Add a web module for the backend code.
a. Click
and select New Web Module. b. Rename the file apiCall.jsw.
c. Paste this code in the module code pane. When called by the frontend, the function in this code retrieves the API key from the Secrets Manager, creates the API request URL, gets the data, and passes it to the frontend for display.
1import { getJSON } from 'wix-fetch';
2import { getSecret } from 'wix-secrets-backend';
3import { getPackageConfig } from 'wix-configs-backend';
4
5const baseUrl = 'https://cloud.iexapis.com/stable/stock/';
6export async function getStockData() {
7 //get the API key
8 const mySecret = await getSecret("stock_API_key");
9 //Build the URL for data retrieval from https://iextrading.com//
10 const sym = await getPackageConfig('defaultSymbol');
11 const fullUrl = `${baseUrl}${sym}/quote?token=${mySecret}`;
12 const quote = await getJSON(fullUrl)
13
14 if (!quote) {
15 console.log('Missing quote in response from API');
16 }
17
18 return quote;
19}
4. Optional best practice: Edit the README.md file to provide documentation about your package.
Learn more in Documenting your Velo Package.
Learn more in Documenting your Velo Package.
Step 3 | Save and Build Your Package
1. Save your package:
a. In the top right corner of Blocks, click Save.
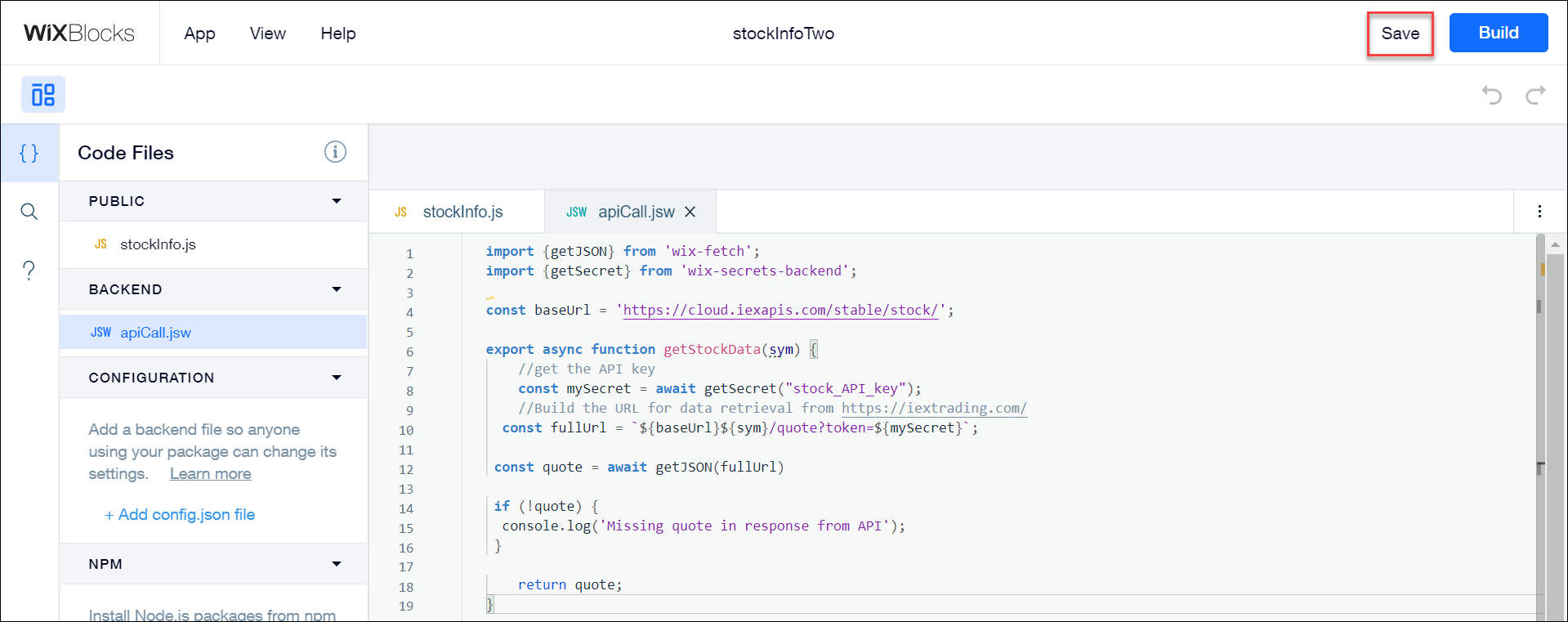
b. Provide the name of your package in the Package Name field. Blocks uses your username and the package name to automatically create an import name for the package. You can modify the import name if you choose.
c. Provide a description of what your package does in the Package Description field. This description appears in the Velo Package Manager.
d. Click Save.
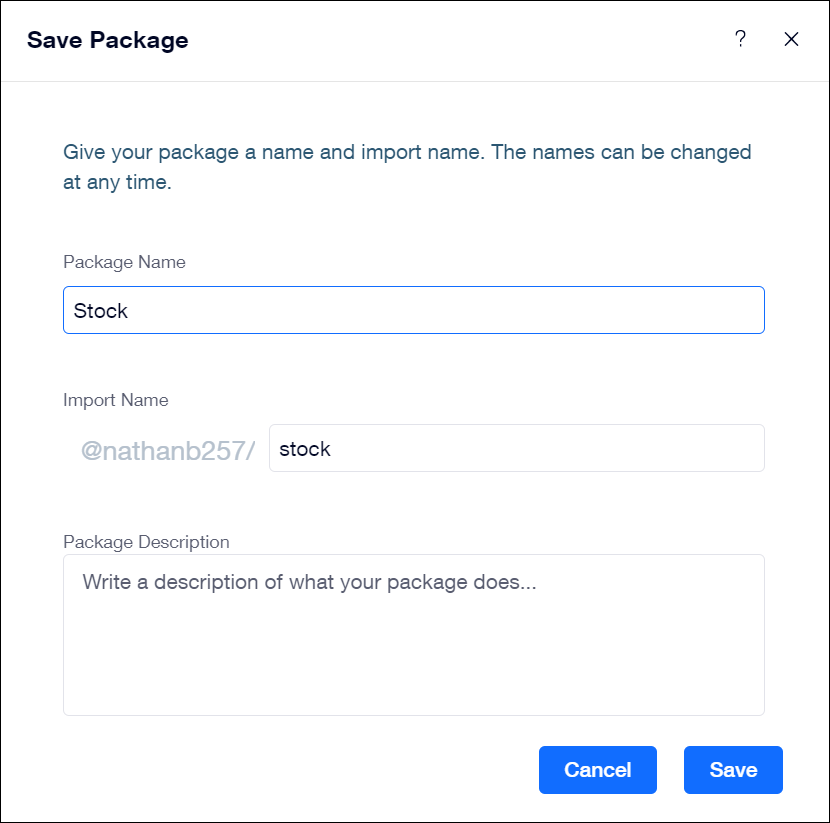
2. Click Build to create a version of your package, so that you can use it on multiple sites.
Step 4 | Use your API calls package
Install your package on your Wix or Editor X sites. You can only use your packages on your own sites.
Work on the site
Perform all of these steps on your Wix or Editor X site (not in Blocks).
- Open your Wix or Editor X Site.
- Make sure that Dev Mode is enabled.
- Click Install Velo Package in the Code Files tab, PACKAGES section.
- Install your package. Note your username and package name in the Code Files tab, PACKAGES section.
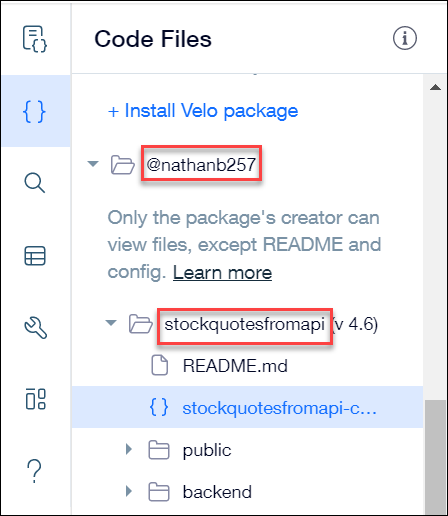
- Store your API key in Secrets Manager with the name stock_API_key.
- Import the getStock() function exported in the public file of the package from the code panel for the page you designed. Be sure to place your username and package name in the code where the placeholders appear.
- Call the getStock() function in onReady().
- Click Preview. When the preview loads, it calls getStock() and the results appear in the console.
1import { getStock } from '@nathanb257/stockquotesfromapi'
2
3$w.onReady(function () {
4 getStock()
5});
6
7
8
9. Change the default symbol in the config.json file, by clicking the file name in your package and typing the new symbol in the code panel that opens.
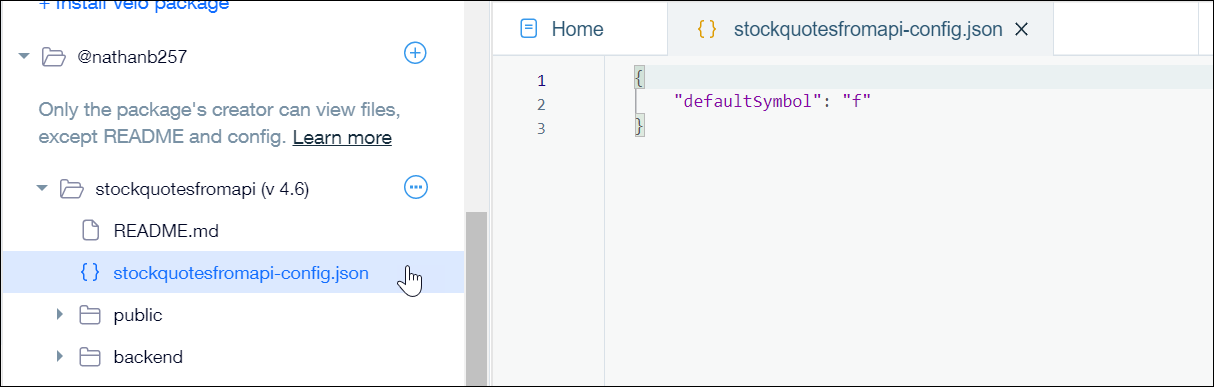
10. Preview the site again to see the returned values for the new symbol.