Velo Example: Coding a Basic Custom Element with Images
4 min
In this article
- What This Article Will Demonstrate
- Code the Custom Element
- Add the Custom Element in the Wix Editor
With Wix custom elements, you can add elements that do not come "out of the box" with WIx. First you create and code the element and its behavior in a JavaScript file. Then add the custom element to your site in the WIx Editor.
This article assumes here you know a bit about designing elements with CSS properties in Javascript and working with web components. This article provides an overview of the code to get you started, providing links and pointers along the way.
What This Article Will Demonstrate
In this article, we'll use this company logo as our custom element:
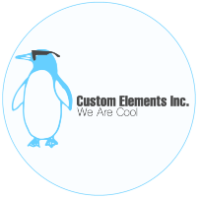
Our goal is to display the custom element on our site, without adding any special behaviors.
Code the Custom Element
Use any IDE to create custom elements in a static Javascript file using the standard ECMAScript 2015 class syntax.
Note: This article provides basic instructions for creating custom elements. For full instructions and examples, see the MDN documentation.
To start, let's code the implementation of a basic, custom element without any special behaviors. For now, this element will be a company logo for display purposes only.
(Our logo comprises two separate images, because we have plans to make the different parts of the logo behave differently. But let's save that for later.)
We assume the logo images have already been uploaded to the Wix Media Manager.
(Our logo comprises two separate images, because we have plans to make the different parts of the logo behave differently. But let's save that for later.)
We assume the logo images have already been uploaded to the Wix Media Manager.
1const LOGO_TXT = 'https://static.wixstatic.com/media/logo-txt.png';
2const LOGO_IMG = 'https://static.wixstatic.com/media/logo-img.png';
Let's add a function that accepts each part of the logo and adds it to the DOM. This function sets the size and position of each logo image.
1const createImg = (id, src) => {
2 const img = document.createElement('img');
3 img.src = src;
4 img.id = id;
5 img.width = '200';
6 img.style.position = 'fixed';
7 return img;
8}
Now we define the actual custom element class.
- The
myLogoDisplay
class is the class of the custom element, which we'll use when registering the custom element. - We use standard
constructor()
andsuper()
functions to create an instance of the element. connectedCallback()
is a lifecycle callback function that is triggered automatically when the element is connected. When the custom element is able to start rendering on the site, the two parts of our logo image can be displayed, with the help of thecreateImg()
function.
1class myLogoDisplay extends HTMLElement {
2 constructor() {
3 super();
4 }
5
6 connectedCallback() {
7 this.appendChild(createImg('txt', LOGO_TXT));
8 this.appendChild(createImg('img', LOGO_IMG));
9 }
10}
Our last step is to register the custom element class with the customElements.define(name, class)
method.
Once registered, new custom elements can be used in your site.
- The first parameter,
my-logo-display
, maps to the tag name, which we'll soon define in the Wix Editor. - The second parameter,
myLogoDisplay
, is the class name of the custom element.
1customElements.define('my-logo-display', myLogoDisplay);
Here is the code for our custom element in a single snippet for your convenience:
1const LOGO_TXT = 'https://static.wixstatic.com/media/logo-txt.png';
2const LOGO_IMG = 'https://static.wixstatic.com/media/logo-img.png';
3
4const createImg = (id, src) => {
5 const img = document.createElement('img');
6 img.src = src;
7 img.id = id;
8 img.width = '200';
9 img.style.position = 'fixed';
10 return img;
11}
12
13class myLogoDisplay extends HTMLElement {
14 constructor() {
15 super();
16 }
17
18 connectedCallback() {
19 this.appendChild(createImg('txt', LOGO_TXT));
20 this.appendChild(createImg('img', LOGO_IMG));
21 }
22
23}
24
25customElements.define('my-logo-display', myLogoDisplay);
Add the Custom Element in the Wix Editor
The next step is to add the custom element to our page.
In the element's Settings:
- Specify the Velo File or Server URL (including the file name in the URL), depending on how you are hosting the JavaScript file.
- Enter the tag name. The tag name corresponds to the element name we registered using the
customElements.define()
function in the previous step. So in this case our tag name ismy-logo-display
. - Save and publish your site.
We have now accomplished our goal, displaying the custom element on our site, without adding any special behaviors.
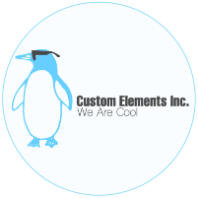
Learn More
Try this example to learn how to change the logo's behavior when visitors perform actions on our site, such as making the logo spin, resize, and shake.