Wix Blocks Tutorial: The Recipes App
18 min read
This tutorial walks you through building the Recipes app. The app showcases recipes on Wix sites, so site visitors can see an image, description and rating for each recipe. They can also subscribe to get new recipes.
The app includes:
- Two widgets - Recipes and Subscribe.
- A custom settings panel.
- A collection for holding the recipes data.
- A Dashboard page for allowing creators to interact with data in the app.
Ready to begin? Create your own copy of the app in Blocks. The link leads to a template with most of the design and some of the code ready.
Note
Throughout this tutorial, you'll move back and forth from creating the app in Wix Blocks to testing it on a Wix site.
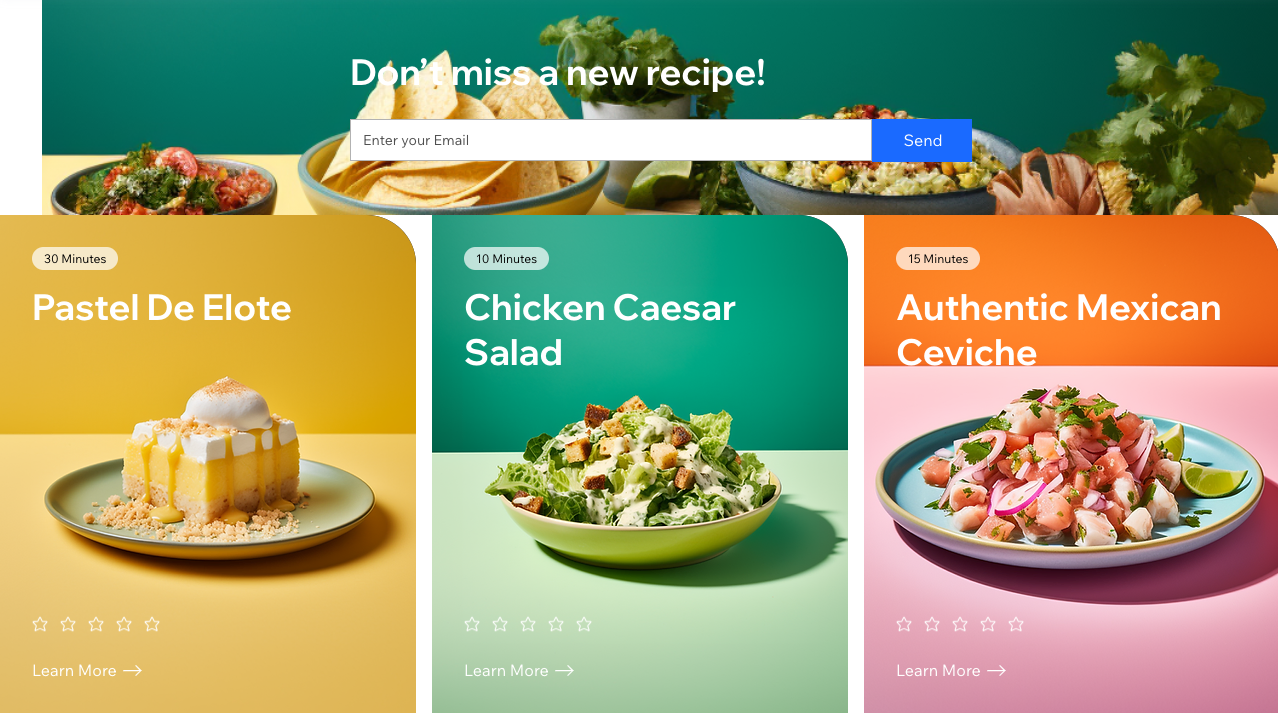
Step 1 | Create a new Design Preset
Design Presets are Block's way of allowing you to have multiple design and layouts for the same widget. They are often used for adapting the app for different devices, like desktop and mobile. The first thing we'll do is create another preset for when the widget is used on a mobile phone. This preset will be a duplicate Card Layout preset, with a few changes.
- In case you missed this first step: click this link to create your own copy of the app.
- Name your app: go to the top bar and click on the
icon next to the app's temporary name.
- Go to the Design Presets menu. The app template already has 3 presets and you'll create a new one now.
- Duplicate the Card Layout preset (to do this, hover, then click the
icon).
- Rename the new preset to Mobile Layout and make sure it is selected.
- Go to the Layout
menu of your widget.
- Change the widget's width to 320px.
- Select your widget's first grid row. Change the first grid row height to 150px.
Tip
Can't see the size of the widget and the grid as presented in the screenshot below? Use the Layers
menu to verify you are selecting the entire widget and not one of its elements.

See screenshot
8. Click the Design
tab.

9. Change the title's font site to 18.
See screenshot
10. Click on the Description, click
and set it to Don't Display, to hide the description in mobile.

See screenshot
11. Go back to the Card Layout preset and check that the description does appear there.
Step 2 | Build the app
Build the app, to create an initial version of it, which you can install on a site. You'll build the app several times during this tutorial.
- Click Build on the top right.
- You will be prompted to create a namespace first. Your namespace allows your app to interact with data collections and code files in its code.
- Build a test version.
Step 3 | Install the app on a site and test it
This steps creates a new Wix site and installs the app on it. Note that installing the app is not enough, you also must add the appropriate widget from the Add
panel.

- In another browser tab, create a new Wix site, or Wix Studio site (you can use wix.new or wixstudio.new).
- Install the app’s test version on that site: click the App Market icon
-> Custom Apps and find your app in the Available Apps.
- The App Widgets section of the Add
panel opens. Click or drag the Recipes widget to add it to your site. There is no need to add the Subscribe widget to the site.
See screenshot
Now let's do some actions on this widget in the site, just to see how it works.
- Click the Stretch
icon to stretch your widget in the site section.
- Go to your desktop
view and change your preset.
- Go to your mobile
view and change your preset again to the Mobile Layout preset.
See in the Wix Editor
See in Wix Studio
4. Right Click on the widget and click Reset Widget, to go back to the original design.
Step 4 | Add the recipes collection in Blocks
Now, go back to Blocks to continue creating the app.
- Go back to Blocks.
- Click the Databases
icon.
- Create a collection called recipes.
- Add the following fields (make sure that the names are exactly the same, no caps):
- image type Image
- description type Text
- duration type Text
- rating type Number
- Go to our demo site, search for the words "Collection data" and save the data file to your computer.
- Import the data to your collection
See screenshot
7. Copy the collection ID
See screenshot
8. Go to collectionUtils.js file in the Public and Backend
files. This is the file for interacting with the app's collection.

9. Change the value of collectionName from '@wixBlocks/recipes-app/recipes' to your collection ID.
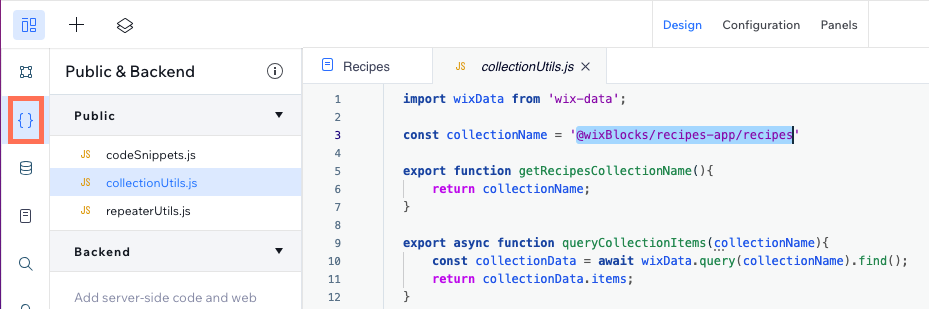
Step 5 | Add logic to the Recipes widget
This step populates the repeater with the data from the collection, using code.
Code snippets
You can also find the following code snippets in the codeSnipets.js file in the Public and Backend
section.

- Click Widgets and Design
to go back to the Recipes widget.
- Paste the following snippet in your widget code.
1import { getRecipesCollectionName, queryCollectionItems } from 'public/collectionUtils'
- Create an initRepeater() function. For now, create the repeater to show 4 items. We'll changes this later.
1async function initRepeater(){
2const collectionItems = await queryCollectionItems(getRecipesCollectionName());
3populateRepeater($w('#recipesRepeater'), collectionItems, 4);
4}
- Await the initRepeater() function in your widget's onReady() function.
1$w.onReady(async function () {
2await initRepeater();
3});
This is the full widget code at the moment:
1// Full Recipes widget's code
2
3 import { populateRepeater } from 'public/repeaterUtils'
4 import { getRecipesCollectionName, queryCollectionItems } from 'public/collectionUtils'
5
6 $w.onReady(async function () {
7 await initRepeater();
8 });
9
10 $widget.onPropsChanged(async (oldProps, newProps) => {
11
12 });
13
14 async function initRepeater(){
15 const collectionItems = await queryCollectionItems(getRecipesCollectionName());
16 populateRepeater($w('#recipesRepeater'), collectionItems, 4);
17 }
18
Step 6 | Create the Recipes widget API
In the previous step we defined the repeater to show 4 items from the database. But what if a site-creator who installed your app wants to show a different number of items? Let's add a property to the Recipes widget API. The property will hold the number of items to display in the widget, and will replace the constant number of 4 that we added in the previous step.
- Click the Widget API
icon, on the right of your widget's code area.
- Click Add New Property
- Name your property itemsToDisplay and give it a display name (such as: "Items to Display", which is more readable).
- Give it a type of number and a default value of 3.
See screenshot
5. Make the populateRepeater function call send the prop as a parameter instead of the 4.
1populateRepeater($w('#recipesRepeater'), collectionItems, $widget.props.itemsToDisplay);
The onPropsChanged() function is triggered when an event of props changing is detected. You can add custom logic to this function.
6. Await the initRepeater() function in your onPropsChanged() function:
1await initRepeater();
Here is the full widget code, after adding the property.
1// Full Recipes widget's code
2
3 import { populateRepeater } from 'public/repeaterUtils'
4 import { getRecipesCollectionName, queryCollectionItems } from 'public/collectionUtils'
5
6 $w.onReady(async function () {
7 await initRepeater();
8 });
9
10 $widget.onPropsChanged(async (oldProps, newProps) => {
11 await initRepeater();
12 });
13
14 async function initRepeater(){
15 const collectionItems = await queryCollectionItems(getRecipesCollectionName());
16 populateRepeater($w('#recipesRepeater'), collectionItems, $widget.props.itemsToDisplay);
17 }
Step 7 | The "My Settings" panel
When a site creator installs your widget, they can change the value of the properties with an autogenerated Settings panel in your widget's floating menu. However, you can also build a custom panel and add custom logic to it.
- Go to your custom panel in the Panels tab. The app has a custom panel names My Settings. Look at the code tab. The code gets the property and sets a slider's value accordingly.
- Add an onChange() function inside the onReady(), so that when the property is changed by the slider, the function notifies the panel to set the widget props again.
1$w('#panelSlider1').onChange(async event => {
2await wixWidget.setProps({itemsToDisplay: event.target.value});
3});
This is the full panel code:
1import wixWidget from 'wix-widget'; // Control your widget's properties, design presets, and more.
2import wixEditor from 'wix-editor'; // interact with the Editor by removing or restoring widget elements, opening Dashboard panels, and more.
3
4$w.onReady(async function () {
5 const props = await wixWidget.getProps();
6 $w('#panelSlider1').value = props.itemsToDisplay;
7
8 $w('#panelSlider1').onChange(async event => {
9 await wixWidget.setProps({ itemsToDisplay: event.target.value });
10 });
11});
Tip
Note the wix-widget and wix-editor modules at the beginning of the panel code. They are unique to Blocks custom panel code.
Step 8 | Make configuration changes
The Configuration tab allows you to control the editor experience of a site creator who installs your app.
- Go to the Configuration tab.
- Click the gray part of the widget, to edit the action bar of the Recipes widget.
- Click the Settings button.
- Click Action Button Settings and connect it to the My Settings panel.
Now, whenever a site creator clicks "Settings", they will see your custom panel.
See screenshot
Another change you can do in the Configuration tab, is prevent layout elements from being selected. A site creator has no reason to select the repeater in your widget.
4. Select the repeater and uncheck Element can be selected box.
See screenshot
Step 9 | Check the app on your site
Let's check that the app works on your site.
- Build a test version of your app in Blocks.
- Go to your Wix editor. Wait to see that the version updated automatically in the Custom Apps panel.
Now let's add data to the collection in the site, to check the app functionality. You need to add data because when a Blocks app is installed on a site, the collection structure is copied to the site, but not the data (learn more).
3. Go to the CMS
and add the CMS to your site.

4. Find your collection.
5. Click on the collection name and open it. See that the default data from Blocks was imported.
See screenshot
6. Click on your widget's Settings button and change the number of items to display.
7. Go to preview and see that the number of items changed. If it did, your test is complete!
Step 10 | Add the Subscription widget
Blocks allows you to create another widget and insert it inside the main widget. For example, let's say that you want to allow people to subscribe to an email list through your Recipes app. To this, we created another widget, named Subscription. However, this is just a dummy widget for the purpose of this tutorial - we didn't implement the code. This is how you add the Subscription widget to your Recipes widget:
- Go to Blocks.
- Open the Add
panel.
- Click My Widgets. Here you can see all the widgets and their presets. Scroll until the end of the list.
4. Drag the Subscribe widget to be on the top part of your Recipes widget.
See screenshot
Step 11 | Make layout changes
Let's make some layout changes to adapt the Subscribe widget to the Recipes widget.
- Click the Subscribe widget (make sure that you select the entire widget and not any internal element).
- Go the Layout
tab of the Inspector
.
- Change the margins to 42px on the top and left.
5. Right click on the Subscribe widget and select Use on All Presets. Now your layout changes apply to all presets.
See screenshot
Let's make some more changes in the mobile preset.
1. Go back to Widgets and Design
and click your Mobile Layout preset.

2. Change the Width to 86%.
3. Align to center - both vertically and horizontally.
See screenshot
Step 12 | Create a Blocks Dashboard Page
Now we want an easy way for users to interact with the collection of recipes, so they can add and manage their recipes. For this, we have the app's Dashboard (learn more). When a site creator installs your app on a site, the Dashboard page will be added with it.
- Go to your app's Dashboard page.
See screenshot
- Let's look at the Dashboard page. The Dashboard is made out of a large multi-state box (you can see this easily in the Layers
panel). The multi-state box has two states, one to manage all the recipes and one to manage individual recipes. You can click on each state and see the difference between them.
See screenshot
- Look at the recipes state. It has two buttons:
- Add Recipe: changes the state of the multi-state box to the form state.
- Manage Collection: navigates to the CMS page in the Dashboard.
- Look at the Dashboard's code tab to see the buttons' code:
1$w('#addBtn').onClick((e) => {
2 $w('#multiStateBox1').changeState('form');
3 })
4
5 $w('#manageCollectionBtn').onClick((e) => {
6 wixDashboard.navigate('wix-databases-lazy-page-component-id');
7 })
Note
wixDashboard.navigate() gives you the ability to navigate to other dashboard pages. You must enable Dev Mode in your site to do so.
- Look at the form state. I has two buttons:
- Cancel cleans the form
- Save uploads the image and updates the repeater.
4. Look at the Dashboard's code tab to see the buttons' code:
1 $w('#cancelBtn').onClick(onCancel)
2 $w('#saveBtn').onClick(onSave);
3
4function onCancel(){
5 $w('#multiStateBox1').changeState('recipes');
6
7 cleanForm()
8}
9
10async function onSave() {
11 let imageUrl = ''
12 if($w('#imageInput').value.length !== 0){
13 const uploadedImage = await $w('#imageInput').uploadFiles();
14 imageUrl = uploadedImage[0].fileUrl;
15 }
16
17 await wixData.insert(getRecipesCollectionName(), {
18 title: $w('#titleInput').value,
19 description: $w('#descriptionInput').value,
20 rating: Number($w('#ratingInput').value),
21 duration: $w('#durationInput').value,
22 image: imageUrl,
23 });
24
25 await initRepeater();
26 $w('#multiStateBox1').changeState('recipes');
27 cleanForm();
28}
29
30async function initRepeater(){
31 const collectionData = await queryCollectionItems(getRecipesCollectionName());
32 populateRepeater($w('#recipesRepeater'), collectionData);
33}
Note
The initRepeater() function in the dashboard code looks almost identical to the initRepeater() in the widget code. They both use the functions from the repeaterUtils.js file.
5. In the Dashboard page, go to Preview and try adding another recipe.
Step 13 | Connect the dashboard to an action button
- Go back to the Recipes widget.
- Go to the Configuration tab.
- Click the secondary action button, which is Change Design.
- Click Action Button Settings.
- Set the button to open a Dashboard instead of a panel.
- Set the main action button text to Manage Recipes instead of Change Design.
See screenshot
Now that we finished building our app, it's time to build a major version (learn more about versions in Blocks).
7. Click Build and select Major Version.
Step 14 | Check the app on your site again
- Go to your site's editor and update the app's version.
See screenshot
2. Open the sites' dashboard.
3. You'll see new dashboard page with your app's name. Click on it.
See screenshot
4. Click Add Recipe and add an item in the dashboard.
5. Click Manage Collection. Open the collection and view the item you just added.
6. You can also go back to the site editor and change the number of items again.
Step 15 | Installation settings
Blocks installation settings determine how this app is installed for other people's websites.
- Go back to Blocks.
- Click WixBlocks -> App.
- Click Installation Settings. A Wix Developers page opens for setting how your app is installed on sites.
- Click the Subscribe widget.
- Set it as Not added automatically (to a site's Add
panel). This is because people will install the main widget and get the inner widget in it.
See screenshot
6. Click the Recipes widget. Set it to be Added to current page.
7. As a default preset, choose the Editorial Layout.
8. Choose the Mobile Layout preset to be the default for mobile.
See screenshot
9. Click Save.
10. Go back to Blocks and click Build again to apply the installation settings.
11. To see the installation settings in action, you need to uninstall and reinstall the app.
Step 16 | Publish your app in the Wix App Market
Blocks apps can be used on your own sites and save you lots of copy-and-paste. They can also be published and sold in the Wix App market and earn money. The last thing we'll do is simulate how to publish your app, in case you want to publish your own app in the future.
- Click WixBlocks -> App.
- Click Publish App. This takes you to Wix Developers.
- Click Pricing. Create a Premium plan and decide on its pricing.
- Build your app in Blocks again.
Now your app has a premium plan. The next step is to configure what happens in Blocks according to the different plans that a user purchased. What extra abilities do you want them to have? For example, in our app, we might want the subscribing option to be disabled until a user paid. Learn more about adapting your app to a pricing plan.
Did this help?
|